In a perfect world, every software developer writes tons of unit tests, and uses continuous integration to make sure everything keeps working. Travis, PHPUnit and Composer are there to save you a lot of time!
In this blogpost I will explain how to setup Travis and run PHPUnit to run unit tests in a PHP project with Composer.
Composer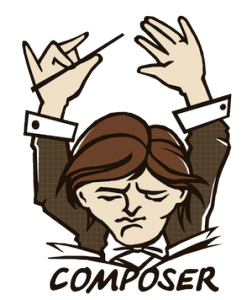
Composer is a super awesome dependency manager for PHP. If you don’t use it yet, you are doing it wrong! 🙂 For this guide I assume you already have a working composer.json
file and you know the basics of Composer.
Before you can actually test something you must of course run composer install
to download all the dependencies.
PHPUnit
PHPUnit is the ultimate unit testing framework for PHP. Installation instructions can be found here.
Next thing to do is create the phpunit.xml
file. This file will contain your PHPUnit configuration and will make it very easy to run the unit tests from the command line.
<?xml version="1.0" encoding="UTF-8"?>
<phpunit backupGlobals="false"
backupStaticAttributes="false"
bootstrap="vendor/autoload.php"
colors="true"
convertErrorsToExceptions="true"
convertNoticesToExceptions="true"
convertWarningsToExceptions="true"
processIsolation="false"
stopOnFailure="false"
syntaxCheck="false"
>
<testsuites>
<testsuite name="My package's test suit">
<directory>./tests/</directory>
</testsuite>
</testsuites>
</phpunit>
In this phpunit.xml
file I have set some basic variables which might be different for your project. But note the bootstrap
argument. Assign the path to your Composer autoload.php
file to the bootstrap
variable.
Next you must specify the directory where your PHP test files are located.
Run your unit tests (and check if PHPUnit is correctly configured): phpunit
This should yield an output like this:
~/S/MyPackage (master) $ phpunit
PHPUnit 4.5.0 by Sebastian Bergmann and contributors.
Configuration read from /Path/To/My/Package/phpunit.xml
.......
Time: 92 ms, Memory: 3.50Mb
OK (7 tests, 24 assertions)
Travis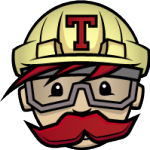
Composer and PHPUnit configured, it’s time to create the .travis.yml
file to configure Travis.
language: php
php:
- 5.4
- 5.5
- 5.6
install:
- composer install --no-interaction
The Travis configuration is just a normal PHP config, but before installing, Travis must run composer install
.
Push everything to Github and let Travis run your unit tests.
If you want strict synchronization between your local Composer dependencies and remote installations, you must also commit the composer.lock
file to your repo. Most people place it in their .gitignore
, but it is actually meant to be committed to Git.
Example
If you need a live example of such a configuration, take a look at my ORM (Github / Travis) package. In this package I specified an other Composer vendor directory (core/lib
), so I also changed the bootstrap
variable in the PHPUnit configuration. You may also ignore the database section in the .travis.yml
file…