Yesterday I had some time left and thought it was the perfect moment to write a very simple live blog to learn more about WebSockets (in Go).
Go
WebSockets are actually very easy in Go, they work just like the http handler. The required websocket package is not installed by default, but you can easily install the (by Go maintained) package: go get code.google.com/p/go.net/websocket
.
Time to create a websocket handler:
func main() {
http.Handle("/", websocket.Handler(HandleSocket))
if err := http.ListenAndServe(":1234", nil); err != nil {
log.Fatal("ListenAndServe:", err)
}
}
In the above snippet, HandleSocket
is the name of your own defined handler function. In this easy example I created a handler function that will just send all received message on a socket back:
func HandleSocket(ws *websocket.Conn) {
// Wait for incoming websocket messages
for {
var reply string
if err := websocket.Message.Receive(ws, &reply); err != nil {
log.Println("Can't receive from socket:", err)
break
}
log.Println("Received back from client: " + reply)
msg := "Received: " + reply
log.Println("Sending to client: " + msg)
if err := websocket.Message.Send(ws, msg); err != nil {
log.Println("Can't send to socket:", err)
break
}
}
}
Of course I enhanced this Go code to create an actual liveblog demo.
Javascript
Now we have our websocket, we must actually do something with it in Javascript. The most simple implementation will prepend any new message to an html list with id messages
:
var sock = null;
var wsuri = "ws://localhost:1234";
window.onload = function() {
sock = new WebSocket(wsuri);
sock.onopen = function() {
console.log("connected to " + wsuri);
}
sock.onclose = function(e) {
console.log("connection closed (" + e.code + ")");
}
sock.onmessage = function(e) {
$('<li>' + e.data '</li>').hide().prependTo('#messages').fadeIn(1000);
}
};
We must also be able to send messages from an admin panel:
function send() {
var msg = document.getElementById('new_message').value;
document.getElementById('new_message').value = '';
sock.send(msg);
};
Liveblog
I combined the above little pieces to create a simple liveblog. The blog has an admin page on which someone can add messages, which are then broadcasted to all the other sockets.
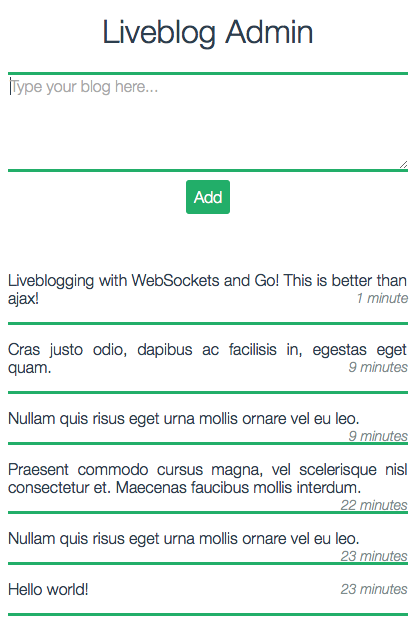
The source code of the demo is available on Github: github.com/DenBeke/Liveblog